Java Program To Reverse The Words In A Sentence
- Java Program To Reverse The Number
- Write A Java Program To Reverse The Sequence Of Words In A Sentence
- Java Program To Reverse Individual Words In A Sentence
I have written a program to reverse the words in a string.if i/p is 'The dog is chasing'then o/p should be 'chasing is dog The'
But I don't know how to write this program using recursion. When I tried searching in stackoverflow, I could find reversing a string; but not reversing the words in a string.
Reverse the Order of the Words in a Sentence in Java Example by Dinesh Thakur Category: Control Structures Stack-This class is a predefined class of java.uti1package.
closed as too broad by John3136, Yvette Colomb♦, Andreas, Ian Stapleton Cordasco, Matt ClarkNov 18 '15 at 4:07
Please edit the question to limit it to a specific problem with enough detail to identify an adequate answer. Avoid asking multiple distinct questions at once. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
Java Program To Reverse The Number
4 Answers
Recursive methods could seem a bit hard when beginning but try to do the following :
Simplify the problem as much as possible to find yourself with the less complicated case to solve. (Here for example, you could use a sentence with two words).
Begin with doing it on a paper, use Pseudocode to help you dealing with the problem with the simplest language possible.
Begin to code and do not forget an escape to your recursion.
Solution
Yassin HajajYassin HajajWrite A Java Program To Reverse The Sequence Of Words In A Sentence
Recursive method, using same logic as linked 'duplicate', without use of split()
: /autorun-virus-remover-s.html.
The logic is:
- Take first char/word
- If that is last char/word, return with it
- Perform recursive call with remaining text (excluding word-separating space).
- Append space (if doing word)
- Append first char/word from step 1
- Return result
Java Program To Reverse Individual Words In A Sentence
As you can see, when applied to reversing text (characters) instead of words, it's very similar:
For people who like things spelled out, and dislike the ternary operator, here are the long versions, with extra braces and support for null values:
Notice how the long version of reverseText()
is exactly like the version in the linked duplicate.
This is a sample program which will do what you want recursively:
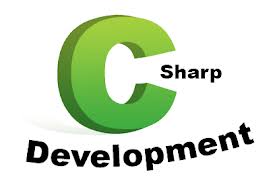
Not the answer you're looking for? Browse other questions tagged javarecursion or ask your own question.
Example: Let the input string be “i like this program very much”. The function should change the string to “much very program this like i”
Examples:
Input: s = “geeks quiz practice code”
Output: s = “code practice quiz geeks”
Input: s = “getting good at coding needs a lot of practice”
Output: s = “practice of lot a needs coding at good getting”
Recommended: Please solve it on “PRACTICE ” first, before moving on to the solution.
Algorithm:
- Initially, reverse the individual words of the given string one by one, for the above example, after reversing individual words the string should be “i ekil siht margorp yrev hcum”.
- Reverse the whole string from start to end to get the desired output “much very program this like i” in the above example.
Below is the implementation of the above approach:
The PCI Express Port Bus Driver is a PCI-PCI Bridge device driver, which attaches to PCI Express Port devices. For each PCI Express Port device, the PCI Express Port Bus Driver searches for all possible services, such as na-tive HP, PME, AER, and VC, implemented by PCI Express Port device. For each service found, the PCI Express Port Bus Driver. Bus driver speed module.
#include <stdio.h> // Function to reverse any sequence // ending with pointer end { while (begin < end) { *begin++ = *end; } void reverseWords( char * s) char * word_begin = s; // Word boundary // explained in the first step temp++; reverse(word_begin, temp - 1); else if (*temp ' ' ) { word_begin = temp + 1; } // Reverse the entire string } // Driver Code { char * temp = s; printf ( '%s' , s); return 0; |

The above code doesn’t handle the cases when the string starts with space. The following version handles this specific case and doesn’t make unnecessary calls to reverse function in the case of multiple space in between. Thanks to rka143 for providing this version.
{ char * temp = s; /* temp is for word boundry */ /*STEP 1 of the above algorithm */ /*This condition is to make sure that the string start with if ((word_begin NULL) && (*temp != ' ' )) { } if (word_begin && ((*(temp + 1) ' ' ) (*(temp + 1) '0' ))) { word_begin = NULL; temp++; reverse(s, temp - 1); |
Time Complexity: O(n)
we can do the above task by splitting and saving the string in reverse manner.
Java
// s = input() { String s[] = 'i like this program very much' .split( ' ' ); for ( int i = s.length - 1 ; i >= 0 ; i--) { } System.out.println(ans.substring( 0 , ans.length() - 1 )); } |
C#
// s = input() using System; { string [] s = 'i like this program very much' .Split( ' ' ); for ( int i = s.Length - 1; i >= 0; i--) { } Console.Write(ans.Substring(0, ans.Length - 1)); } |
Python3
# s = input() words = s.split( ' ' ) for word in words: print ( ' ' .join(string)) # Solution proposed bu Uttam |
Please write comments if you find any bug in above code/algorithm, or find other ways to solve the same problem.
Recommended Posts:
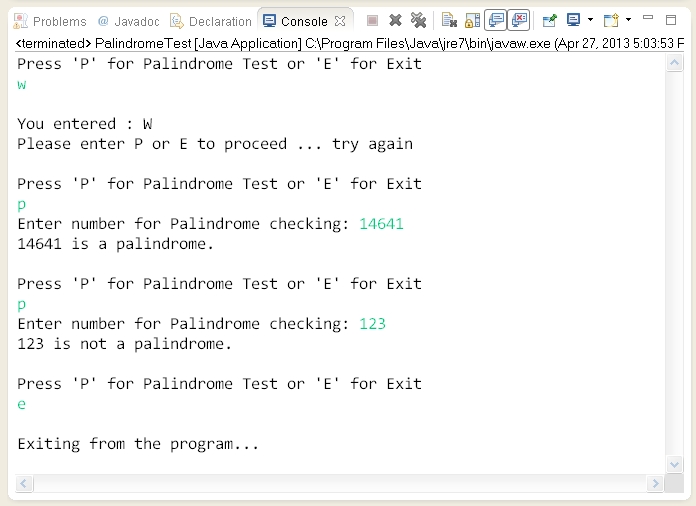
Improved By : Uttamk94, Deepak Kumar Agarwal, Ita_c